Vue Js Create Array of Object Dynamically:In Vue.js, you can create an array of objects dynamically by using the built-in array method called push()
. The push()
method allows you to add new objects to the end of an existing array. To create a new object dynamically, you can define it as a JavaScript object literal and then push it into the array using the push()
method. For example, suppose you have an empty array called items
and you want to add a new object with properties name
and price
. You can create the object { name: "item1", price: 10 }
and push it into the items
array using items.push({ name: "item1", price: 10 })
. This will dynamically create and add a new object to the array.
How can you dynamically create an array of objects in Vue js?
In the Below code, the array of objects is already dynamically created in the Vue instance’s data
property with an initial value of an empty array []
. To add new objects to the array dynamically based on user input, the addObject()
method is used. This method creates a new object using the values entered by the user in the input fields, pushes it to the objects
array, and then clears the input fields.
This approach allows for dynamic creation of an array of objects as the user can add as many objects as they want by clicking the “Add Object” button, and each time a new object will be created and added to the array.
Therefore, to create a new object dynamically, you can follow the example above and create a new object using the values entered by the user in the input fields, push it to the array using the push()
method, and then clear the input fields.
Vue Js Create Array of Object Dynamically Example
<div id="app">
<form @submit.prevent="addObject">
<div>
<label>Name:</label>
<input v-model="name" required>
</div>
<div>
<label>Age:</label>
<input v-model.number="age" type="number" required>
</div>
<div>
<button type="submit">Add Object</button>
</div>
</form>
<ul>
<li v-for="(obj, index) in objects" :key="index">
Object {{ index + 1 }} - Name: {{ obj.name }}, Age: {{ obj.age }}
</li>
</ul>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
objects: [], // initial empty array
name: '',
age: ''
};
},
methods: {
addObject() {
// create a new object with input values
const newObj = {
name: this.name,
age: this.age
};
// add the object to the array
this.objects.push(newObj);
// clear the input fields
this.name = '';
this.age = '';
}
}
});
</script>
Output of Vue Js Create Array of Object Dynamically
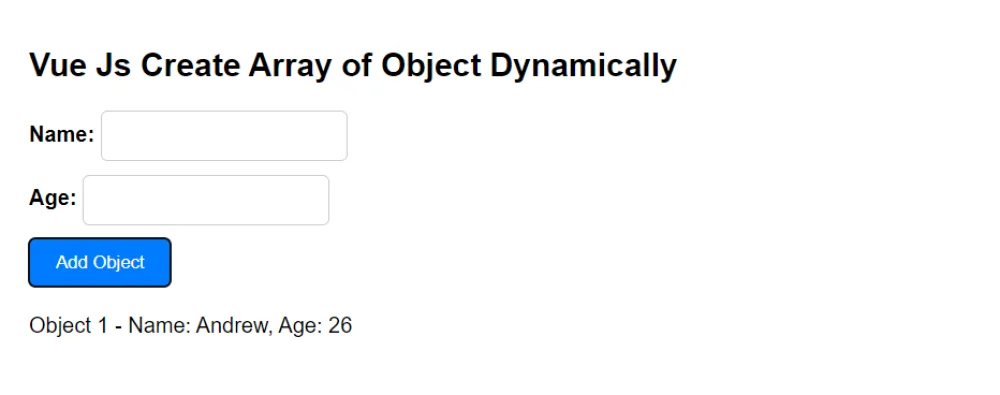